Bubble Sort, Insertion Sort, Selection Sort, Quick Sort, Merge Sort, and Tim Sort.
Tim Sort:
Timsort is a hybrid sorting algorithm, derived from merge sort and insertion sort, designed to perform well on many kinds of real-world data. It was invented by Tim Peters in 2002 for use in the Python programming language. The algorithm finds subsets of the data that are already ordered, and uses that knowledge to sort the remainder more efficiently. This is done by merging an identified subset, called a run, with existing runs until certain criteria are fulfilled. Timsort has been Python's standard sorting algorithm since version 2.3. It is used to sort arrays of non-primitive type in Java SE 7 on the Android platform and in GNU Octave.
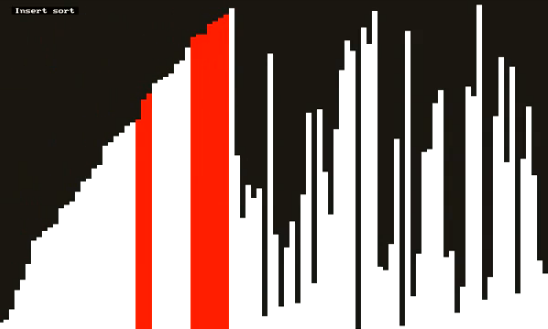
And the graph below shows the efficiency comparison of the Selection Sort, Merge Sort, Intersection Sort [, and Array Sort]

From the Graph one can see that selection sort is the least efficient one compared to the three others. For insertion sort and merge sort, is better to chose Merge Sort for a longer list as it grows more liner than Insertion sort. Overall, Arrays Sort is the best one out of this four types.
When talking about sorting algorithms, we usually look at the best case, worst case and average case. And this bring us to Big O. For example, best case for insertion sort is O(n), average case is O(n**2), and worst case is O(n**2); and other example, best case is O(n log n) for typical and O(n) for natural variant, average case is O(n log n), worst case is O(n log n).